How to Copy to and from the Clipboard using C#
Hey everyone and welcome back! Today we will be making a C# copy to clipboard style application to learn how to use the C# Clipboard library!
Today we’re going to be learning about the clipboard, which is a really cool thing to be able to use in C#! On top of being a super useful thing to manipulate, the clipboard is really easy to work with!
If you aren’t familiar with the clipboard, what I’m talking about is when you type a word and then right-click and then copy the text. This copies the text to your “Clipboard”
The Clipboard is kind of an invisible notepad that you can copy things to and paste from. For example, you could type your name out, copy your name and then paste it to multiple places. This saves you from typing the same thing over and over again.
Alright! On to the programming! We can easily access this clipboard and have our programs do some pretty cool stuff!
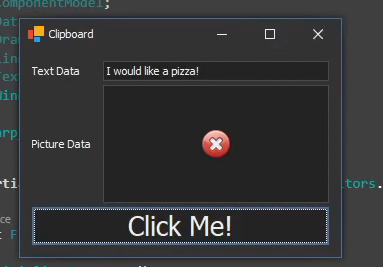
First, you are going to want to create a sample application like the one I have above (also shown in the video)
This little form has a text box at the top, a picture box in the middle and a button on the bottom. Nothing special there. Next, we are going to go ahead and add some code.
Double click on the button and that will create an event handler for the button’s click event. This is where we are going to do most of our operations.
To access the clipboard we are going to use the clipboard class that is inside of the system.windows namespace. So the first thing to do is make sure system.windows is imported.
using System.Windows;
Once you have system.windows imported, it’s time to use the clipboard.getText function to get the text currently saved to your clipboard.
this.txtGetData.Text = Clipboard.GetText();
Our textbox is named txtGetData and this line will set the text of that textbox to whatever text is saved in your clipboard using the Clipboard.GetText function!
In the video, I wrote some text out in notepad and copied it. Once the text was in my clipboard, all I had to do was click the giant button at the bottom to run the code and have it set the textbox’s text to what I had copied.
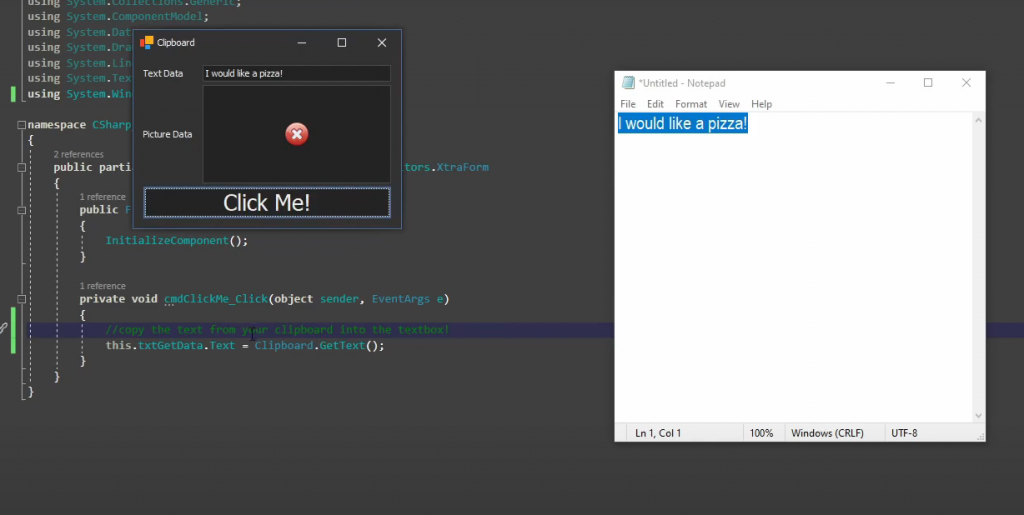
The next function we are going to use is the Clipboard.GetImage() function. This can be used to retrieve an image that has been copied to your clipboard. My picturebox is named pbData and the line of code to copy the image from the clipboard looks like this:
pbData.Image = Clipboard.GetImage();
So for our example, I am going to pull up a random image using google images and then copy it to my clipboard by pressing the button.
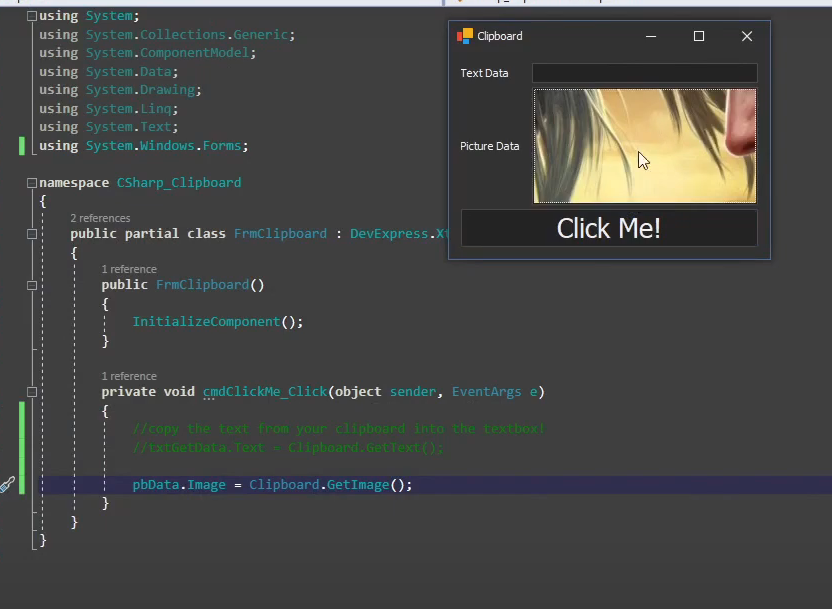
Next, we are going to write some text TO our clipboard. This is done in a very similar way. Instead of GetText() we are going to use SetText(). Below is an example of me setting the text of my clipboard to whatever was typed into the textbox.
Clipboard.SetText(txtGetData.Text);
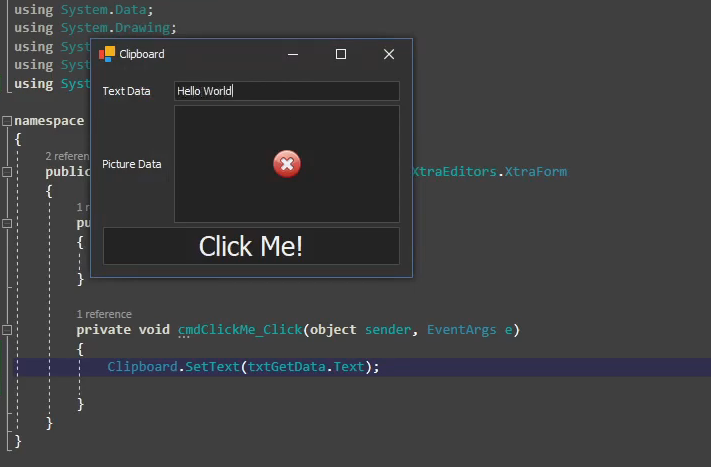
Copying an image to your clipboard is done in a very similar way. The code for that (with a picture box named pbData) is:
Clipboard.SetImage(pbData.Image);
And… That’s about it for copying text & images to and from the clipboard! Those are definitely the most common uses for the Clipboard class but there are two more relatively common things you can use the Clipboard class for. You can copy raw Data to and from the clipboard and you can clear the clipboard.
To copy raw data from the clipboard, you are going to use this function:
Object obj = Clipboard.GetData();
This can be used to copy/paste all kinds of data!
The last item on our list to cover is the clear function. This will erase any data that is currently stored in your clipboard!
Clipboard.Clear();
And that about wraps up working with the Clipboard in C#! I hope this helped you on your current project and thanks for reading!