When trying to send an email via SMTP you may get the dreaded “The SMTP server requires a secure connection or the client was not authenticated” error. This error is annoying but is actually really easy to fix!
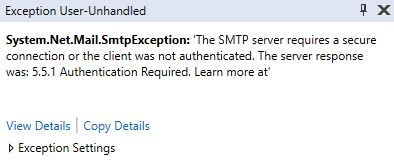
Why did I receive the SMTP server Authentication error?
This authentication error actually happens because Google is blocking you from signing into your Gmail account. The reason for this used to be a setting called “Allow less secure apps.” but now you will have to generate an App Password and use that in your code.
Google doesn’t initially allow any application to log into your Google account. You will have to give permission for applications to access your Gmail account. This used to require a simple setting change to allow unsecured applications. Google has since changed that policy and you will have to generate a password for your application. I will show you how to do that and include an example code below!
How to fix “The SMTP server requires a secure connection or the client was not authenticated” – Old Method
Previously, To fix this error, we simply need to enable the following setting in your google account: “Allow less secure apps.” You will see this method suggested a lot all over the internet but it does not work anymore. I will provide the new solution below!
Old Method to enable “Allow less secure apps.”:
- Login to your Gmail Account
- Navigate to the security settings for your Google Account
- Navigate to “Less secure App Access” and turn on “Allow less secure apps”
Once you click the toggle switch and it has been enabled, your app should now be working, or at least… It would have before the latest Google update! Previously this would have fixed your authentication issue but now you just receive this error message.
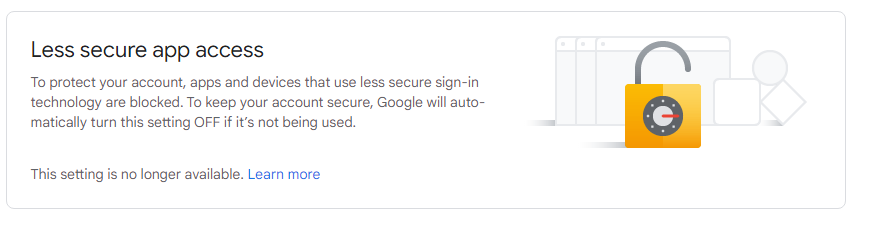
Google has actually disabled this super easy fix as of May 30th 2022. Now we have to generate a unique password for our application, which is thankfully really easy to do!
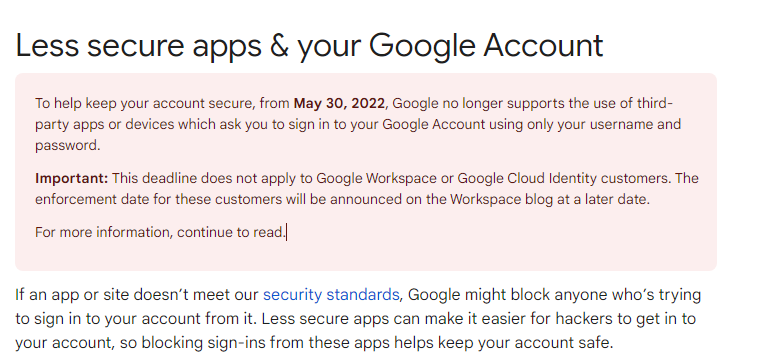
How to fix “The SMTP server requires a secure connection or the client was not authenticated” – after May 30th 2022
Google has now disabled the ability to enable the use of less secure applications. Instead, we will be getting an App Password and using that in our application. Follow the steps below to get your app password and then we will discuss how to use it in your application with an example.
Generate an App Password to fix the SMTP client not authenticated issue
- Log in to your Google account
Navigate to Gmail.com and log in with your Google account. After that, click on “Manage your Google account”
- Navigate to the security section of your Google account
Click on “Security” in the menu on the left
- Search for “App Passwords” in the top search box.
Navigate to the App Passwords page by searching in the search box at the top of the page.
- Generate a new App Password
Once on the App passwords screen, click “Select App” and choose “Mail” and then for the “Select Device” drop down, choose “Windows Computer”. After you have those options selected, click the Generate button.
- Use your new App Password
Now that you have your app password, it is time to use it in our application to fix the client was not authenticated error message!
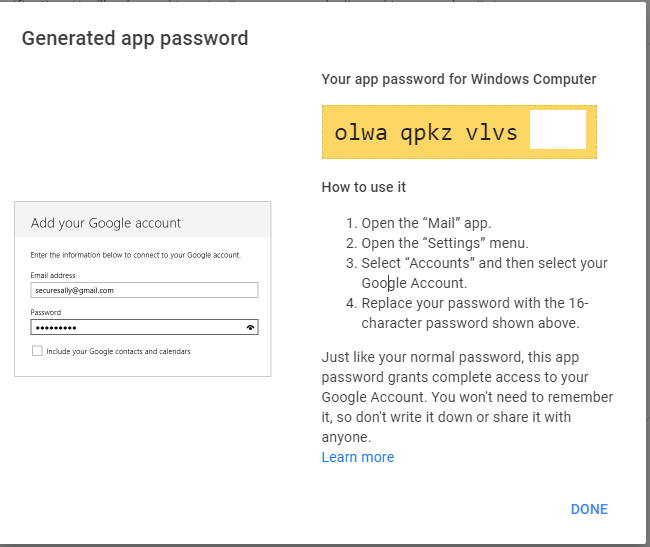
Example code to send an email using the new app password:
using System;
using System.Net;
using System.Net.Mail;
namespace GmailSmtpConsoleApp
{
class Program
{
private const string ToAddress = "testemail@testing.com";
private const string FromAddress = "testemail@testing.com";
private const string GoogleAppPassword = "XXXXXXXX";
private const string EmailSubject = "Test email!!";
private const string EmailBody = "<h1>Hi</h1>";
static void Main(string[] args)
{
Console.WriteLine("Hello World!");
var smtpClient = new SmtpClient("smtp.gmail.com")
{
Port = 587,
Credentials = new NetworkCredential(FromAddress , GoogleAppPassword),
EnableSsl = true,
};
var mailMessage = new MailMessage
{
From = new MailAddress(FromAddress),
Subject = EmailSubject,
Body = EmailBody,
IsBodyHtml = true,
};
mailMessage.To.Add(ToAddress);
smtpClient.Send(mailMessage);
}
}
}
This code should be pretty straightforward, we are just passing our application password to the Network Credential object that we made. We then pass that NetworkCredential object off to Gmail when we send our message.
Thanks for reading and I hope this helped you fix your “The SMTP server requires a secure connection or the client was not authenticated” error message! If you are still having problems, feel free to ask any questions in the comments below and I would be happy to help!