How to write a New Line in C#
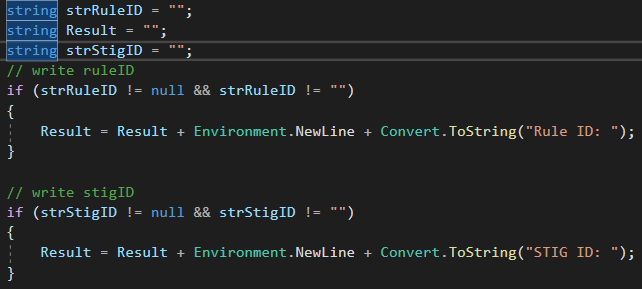
Today we are going to discuss how to write a New Line in C#! This is a simple question but the answer changes based on what you are working on. If you are creating a console application, something like Console.Writeline(); will work but if you are creating a string, you will need something like “\n”. So with that being said, let’s dive right in!
How to write a new line in a console application
To write a new line inside of a console application in C# you can use Console.Writeline. This is really easy to use since you will just write a blank line out before or after your other lines. I’ll give an example below.
Console.Writeline("Apples");
Console.Writeline(); //This is our new line
Console.Writeline("Oranges");
With the above code, you will be able to see the new line between apples and Oranges. If you are creating a console application, that is all there is to it!
How to write a New Line inside of a string in C#
When you want to write a new line inside of a string, you can use “\n”. This will cause whatever is interpreting the string to add a line break where the “\n” is located. This can be handy when sending text to a rich textbox or saving a newline character to a database. Below is a code example using “\n” to add new line into a string in C#
Console.WriteLine("This is the first line.\nThis is the line after.");
The simple way to write a new line in C#
The easiest way to write a new line in C# is to use the Environment.Newline method. This method is super simple, it’s just like the above method with “\n” but instead you append Environment.Newline to the string! This inserts a special character that Rich textboxes and the console will read as a new line.
Below you can see a code example with the usage for Environment.Newline. This is the method I use the most because of how simple it is. This makes it really easy for other people to know what you are trying to do when they read your code.
Console.WriteLine("This is the first line." + Environment.NewLine + "This is the second line.");
This method for writing a new line will work in a console application or in a windows forms application.
Wrapping up
I hope this helps you add line breaks to your C# applications. This method works almost identically in VB.Net as well. The concept it’s self works the same in PHP and many other languages. Thanks for reading and I’ll see you in the next one!